programming — Thu Jun 10 2021
Build better React apps with NextJS
Post by — Joy Krinbut
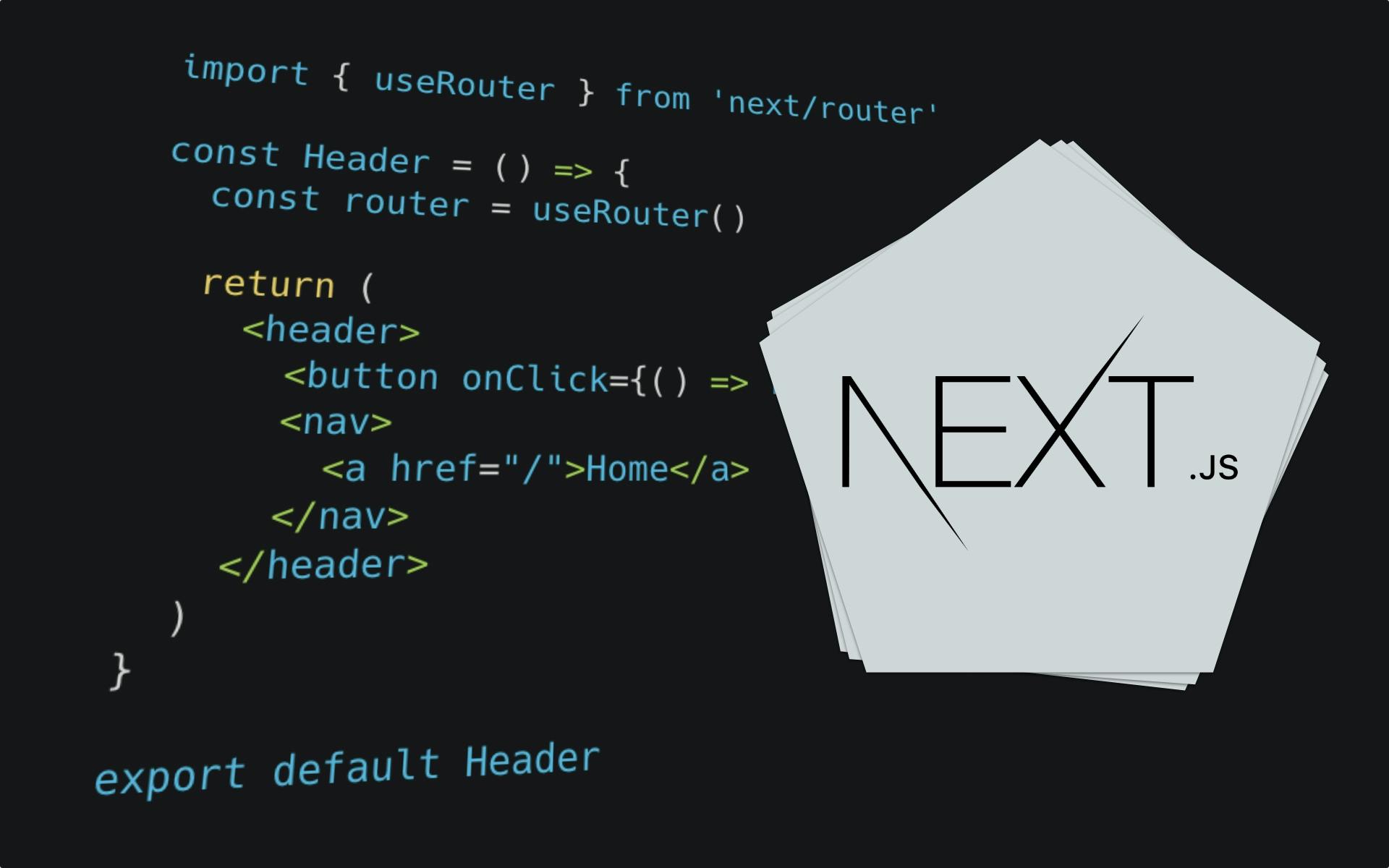
Many developers are consumers of the React framework but when we need to go beyond just creating frontend React nextJS comes in handy. In this article we cover NextJS, what it is and how we can better React apps with NextJS.
What is NextJS?
Next.js is a React front-end framework(meaning it builds on the react framework) that lets you optimize performance and user experience through additional pre-rendering features like out-of-the-box server-side rendering and static site generation. It was created by a hosting company called Vercel(formally called Zeit). You don’t need any configuration of webpack or similar tools to start using Next.js. It comes preconfigured for production. It is also called the framework for production. Next.js is used by full-stack developers to build reactive websites as it requires a good understanding of both client-side React and server-side architectures.
Please Note, This post assumes that you have at least basic knowledge of React and JavaScript.
Server Side Rendering(SSR)
The section above mentioned that NextJS implements sever side rendering. Which is a render technique used by NextJS and also traditional applications. In SSR the server is in charge of render view to page, unlike like client side rendering where the view is render by client side JavaScript. SSR allows the server to access all required data and process the JavaScript together to render the page. Then, the page is sent back in its entirety to the browser and immediately rendered. SSR allows webpages to load in a fraction of the time and increases user experience with added responsiveness.
Static Site Generation
This is the process of compiling and rendering a website or web app at build time. The output is a bunch of static files, including the HTML file itself and assets like JavaScript and CSS. Essentially, a static site generator automates the task of coding individual HTML pages and gets those pages ready to serve to users ahead of time.
Why should use NextJS?
There are reasons why you should consider using NextJS
- Search Engine Optimization (SEO)
Because NextJS use SSR and Static Site Generation to render pages, apps built with NextJS are extremely fast and efficient by default, thereby improving SEO and User Experience. NextJS prerenders pages on the server which makes it very easy for search engines and bots to easy scrape data on the website. - Pre-rendering, both static generation (SSG) and server-side rendering (SSR) are supported
Server-side rendering (SSR) is preparing of content of a page on a server, while one-page React application uses client-side rendering (CSR). With SSR you can easily make async actions before the page loads. I.e Imagine you have a backend API and your NextJS app on different servers (off course with NextJS you can couple them together), you can easily fetch data like user data before the page ever finish loading. All this can be done without the need of a loader because it would be done before the page renders. - Maintains the benefits of React such as intuitive component-driven development and front-end state system
NextJS is still React with added features, so all react features are still applicable. - Full-stack capabilities
NextJS makes it easier for React developers to add back-end code to the project. It very easy here to add our own code for storing data, getting data, authentication etc. - Automatic code splitting
Next.js is clever enough to only load the Javascript and CSS that are needed for any given page. This makes for much faster page loading times, as a user’s browser doesn't have to download Javascript and CSS that it doesn't need for the specific page the user is viewing. This increases performance as there is less for the user’s browser to download and the user benefits from seeing the page content quicker. - Hot Module Replacement (HMR)
This is less important for the end users of an application but very important for developers. HMR allows developers to see any changes they have made during development, live in the application as soon as they have been made. However, unlike traditional "live reload" methods, it only reloads the modules that have actually changed, preserving the state the application was in and significantly reducing the amount of time required to see changes in action.
Getting Started
Getting started with NextJS is very easily. Just like React, create-react-app can easily get you started like-wise NextJS with create-next-app.
Open your terminal and install create-next-app globally, run this command
npm install create-next-app -g
After that finished, open the directory where you want project and run this command
create-next-app <app-name>
Then open the project folder with favorite code editor and start developing. You add edit the default pages or add pages by creating files in pages folder. Check out the NextJS docs for more.